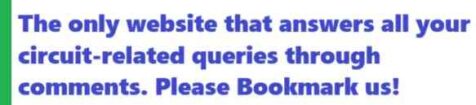
In this post we are going to construct a circuit which can measure the speed of any vehicle on roads and highways. The proposed circuit is kept stationary at a place where the vehicles are suspected to over-speed. If any vehicle goes beyond the speed limit, the circuit alerts immediately. We will be looking the code, circuit diagram and logic how the speed of the vehicle is measured.
Objective
Over speeding causes 75% road accidents according to accidental death report 2015 in India, that’s a huge number. Most traffic police tries to detain the motorists who dangerously drive their vehicle beyond city speed limit.
Not every time a traffic police can stop an over speeding vehicle and charge them. So a device called speed camera is installed where the motorists are suspected to over speed such as frequent accident prone areas, intersections etc.
We are going to build something similar to speed camera, but in a much simplified way, which can be installed inside a campus such as school, college or IT parks or just as a fun project.
The proposed project consists of 16 x 2 LCD display to showcase the speed of each vehicle passing through; two laser beams which are placed apart exactly 10 meters to measure the speed of the vehicle while interrupting those laser beams.
A buzzer will beep when a vehicle is passed; indicating that a vehicle is detected and the speed of each vehicle will be displayed on the LCD display. When a vehicle is going beyond the speed limit the buzzer will beep continuously and speed of vehicle will be shown on the display.
NOTE: Speed of the vehicle will be displayed on LCD regardless of the vehicle is going over speed or under speed.
Now let’s see the logic behind the circuit for measuring speed.
We all know a simple formula called speed – distance – time formula.
Speed = Distance / Time.
• Speed in meter per second,
• Distance in meter,
• Time in seconds.
To know the speed, we have to know the distance say “x” traveled by a vehicle and time taken to cover that distance “x”.
To do this we are setting up two laser beams and two LDRs with 10 meter distance in the following fashion:
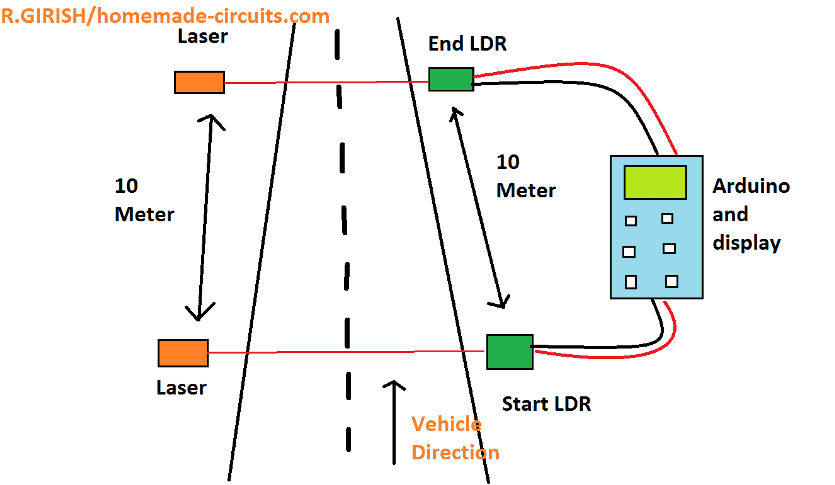

We know the distance is 10 meter which is fixed, now we have to know the time in the equation.
The time will be calculated by Arduino, when the vehicle interrupts the “start laser”, the timer begins and when the vehicle interrupts the “end laser” the timer stops and applying the values to the equation Arduino will find the speed of the vehicle.
Please note that speed of the vehicle will only be detected in one direction i.e. start laser to stop laser, to detect the vehicle in another direction another same setup has to be place on opposite direction. So, this is ideal for places like school, collage etc. where they have IN and OUT gates.
Now let’s see the schematic diagram:
Connection between Arduino and display:
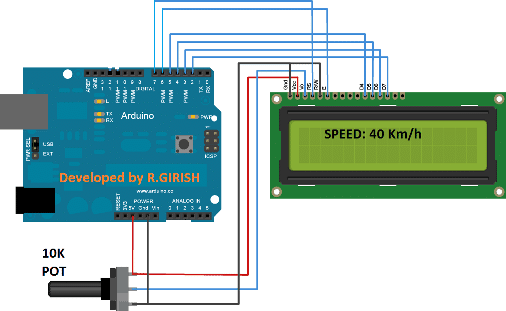
There above circuit is self-explanatory and just connect the wiring as per the circuit. Adjust the 10K potentiometer for adjusting the display contrast.
Additional Wiring Details:
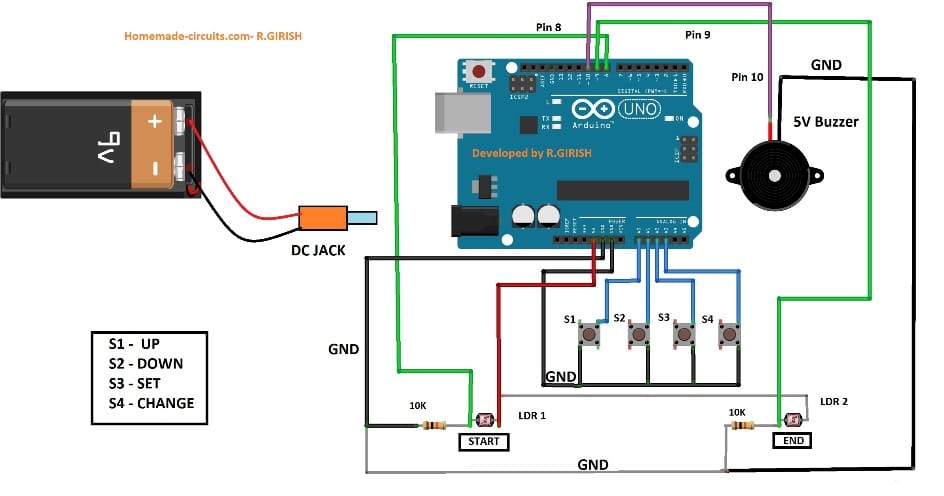
The above circuit consists of Arduino, 4 push buttons, two 10K pull down resistors (don’t change the value of resistors), two LDRs and one buzzer. The function of 4 push buttons will be explained shortly. Now let’s see how to mount the LDR properly.

The LDR must me covered from the sunlight properly, only the laser beam should strike the LDR. Make sure that your laser module is powerful enough to work in a bright sun shine.
You can use a PVC pipe for the above purpose and paint it black inside the tube; don’t forget to cover the front part, use your creativity to accomplish this.
Program Code:
// ----------- Developed by R.GIRISH ---------//
#include <LiquidCrystal.h>
#include<EEPROM.h>
const int rs = 7;
const int en = 6;
const int d4 = 5;
const int d5 = 4;
const int d6 = 3;
const int d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
const int up = A0;
const int down = A1;
const int Set = A2;
const int change = A3;
const int start = 8;
const int End = 9;
const int buzzer = 10;
const float km_h = 3.6;
int distance = 10; // In meters.
int variable = 0;
int count = 0;
int address = 0;
int value = 100;
int speed_address = 1;
int speed_value = 0;
int i = 0;
float ms = 0;
float Seconds = 0;
float Speed = 0;
boolean buzz = false;
boolean laser = false;
boolean x = false;
boolean y = false;
void setup()
{
pinMode(start, INPUT);
pinMode(End, INPUT);
pinMode(up, INPUT);
pinMode(down, INPUT);
pinMode(Set, INPUT);
pinMode(change, INPUT);
pinMode(buzzer, OUTPUT);
digitalWrite(change, HIGH);
digitalWrite(up, HIGH);
digitalWrite(down, HIGH);
digitalWrite(Set, HIGH);
digitalWrite(buzzer, LOW);
lcd.begin(16, 2);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print(F(" Vehicle Speed"));
lcd.setCursor(0, 1);
lcd.print(F(" detector"));
delay(1500);
if (EEPROM.read(address) != value)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Set Speed Limit");
lcd.setCursor(0, 1);
lcd.print("km/h:");
lcd.setCursor(6, 1);
lcd.print(count);
while (x == false)
{
if (digitalRead(up) == LOW)
{
lcd.setCursor(6, 1);
count = count + 1;
lcd.print(count);
delay(200);
}
if (digitalRead(down) == LOW)
{
lcd.setCursor(6, 1);
count = count - 1;
lcd.print(count);
delay(200);
}
if (digitalRead(Set) == LOW)
{
speed_value = count;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Speed Limit is");
lcd.setCursor(0, 1);
lcd.print("set to ");
lcd.print(speed_value);
lcd.print(" km/h");
EEPROM.write(speed_address, speed_value);
delay(2000);
x = true;
}
}
EEPROM.write(address, value);
}
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Testing Laser");
lcd.setCursor(0, 1);
lcd.print("Alignment....");
delay(1500);
while (laser == false)
{
if (digitalRead(start) == HIGH && digitalRead(End) == HIGH)
{
laser = true;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Laser Alignment");
lcd.setCursor(0, 1);
lcd.print("Status: OK");
delay(1500);
}
while (digitalRead(start) == LOW && digitalRead(End) == LOW)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Both Lasers are");
lcd.setCursor(0, 1);
lcd.print("not Aligned");
delay(1000);
}
while (digitalRead(start) == LOW)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Start Laser not");
lcd.setCursor(0, 1);
lcd.print("Aligned");
delay(1000);
}
while (digitalRead(End) == LOW)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("End Laser not");
lcd.setCursor(0, 1);
lcd.print("Aligned");
delay(1000);
}
}
lcd.clear();
}
void loop()
{
if (digitalRead(change) == LOW)
{
change_limit();
}
if (digitalRead(start) == LOW)
{
variable = 1;
buzz = true;
while (variable == 1)
{
ms = ms + 1;
delay(1);
if (digitalRead(End) == LOW)
{
variable = 0;
}
}
Seconds = ms / 1000;
ms = 0;
}
if (Speed < EEPROM.read(speed_address))
{
y = true;
}
Speed = distance / Seconds;
Speed = Speed * km_h;
if (isinf(Speed))
{
lcd.setCursor(0, 0);
lcd.print("Speed:0.00");
lcd.print(" km/h ");
}
else
{
lcd.setCursor(0, 0);
lcd.print("Speed:");
lcd.print(Speed);
lcd.print("km/h ");
lcd.setCursor(0, 1);
lcd.print(" ");
if (buzz == true)
{
buzz = false;
digitalWrite(buzzer, HIGH);
delay(100);
digitalWrite(buzzer, LOW);
}
if (Speed > EEPROM.read(speed_address))
{
lcd.setCursor(0, 0);
lcd.print("Speed:");
lcd.print(Speed);
lcd.print("km/h ");
lcd.setCursor(0, 1);
lcd.print("Overspeed Alert!");
if (y == true)
{
y = false;
for (i = 0; i < 45; i++)
{
digitalWrite(buzzer, HIGH);
delay(50);
digitalWrite(buzzer, LOW);
delay(50);
}
}
}
}
}
void change_limit()
{
x = false;
count = EEPROM.read(speed_address);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Set Speed Limit");
lcd.setCursor(0, 1);
lcd.print("km/h:");
lcd.setCursor(6, 1);
lcd.print(count);
while (x == false)
{
if (digitalRead(up) == LOW)
{
lcd.setCursor(6, 1);
count = count + 1;
lcd.print(count);
delay(200);
}
if (digitalRead(down) == LOW)
{
lcd.setCursor(6, 1);
count = count - 1;
lcd.print(count);
delay(200);
}
if (digitalRead(Set) == LOW)
{
speed_value = count;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Speed Limit is");
lcd.setCursor(0, 1);
lcd.print("set to ");
lcd.print(speed_value);
lcd.print(" km/h");
EEPROM.write(speed_address, speed_value);
delay(2000);
x = true;
lcd.clear();
}
}
}
// ----------- Developed by R.GIRISH ---------//
Now let’s see how to operate this circuit:
• Complete you circuit and upload the code.
• The distance between two lasers / LDRs should be exactly 10 meter, no less or no more, Otherwise speed will be miscalculated (shown in the first diagram).
• The distance between the laser and LDR can of your choice and circumstances.
• The circuit will check for laser misalignment with LDR, if any please correct it as per the information displayed on the LCD.
• Initially the circuit will ask you to enter a speed limit value in km/h beyond which the circuit alerts, by pressing up (S1) and down (S2) you can change the number on the display and press set (S3), this value will be saved.
• To change this speed limit, press button S4 and you can set a new speed limit.
• Now take a motor bike drive at 30 km/h and interrupt the laser beams, the circuit should show you a number very close to 30 km/h.
• You are done and your circuit is ready to serve your campus safety.
Author’s prototype:
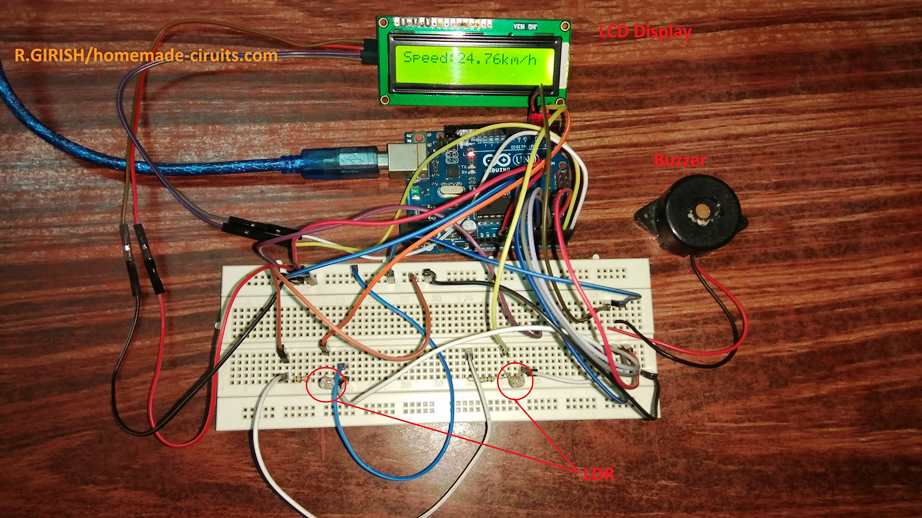
If have any questions regarding this traffic police vehicle speed detector circuit, please feel free to ask in comment section, you may get a quick reply.
Have Questions? Please Comment below to Solve your Queries! Comments must be Related to the above Topic!!