In this post I will show how to construct an automated water irrigation system for small garden using arduino and soil moisture sensor.
Introduction
The proposed system can monitor the soil moisture level and when soil moisture goes below preset value, the 12V DC pump will be triggered for predetermined period of time. The status of the soil moisture level and other functions of the system can be monitored via 16 x 2 LCD display in real time.
It is estimated that there are 3 trillion trees across the globe which is greater than the number of start in our home Milky Way galaxy which is estimated to be 100 billion. But, we humans cut countless number of trees to fulfil our basic needs to luxury needs.
Mother Nature is designed with a feedback system, when a species introduces huge disturbances, nature will wipe the species out of existence.
Human beings were disturbing the nature unknowingly for centuries but, even after great development in science and technology the rate of disturbance haven’t reduced.
Climate change is one of the examples, when it gets drastic enough our species won’t last long.
This project take a baby step forward to preserve the nature, it can irrigate your lovely small garden without any human interaction. Now let’s get in to technical details of the project.
Soil Moisture Sensor:
The heart of the project is soil moisture sensor which can sense the amount of moisture content in soil. The sensor gives out analog value and a microcontroller will interpret those values and display the moisture content.
There are two electrodes, which will be inserted in the soil. The electrodes are connected to a circuit board consisting of comparator IC, LED, trimmer resistor input and output pins.
Illustration of soil moisture sensor:
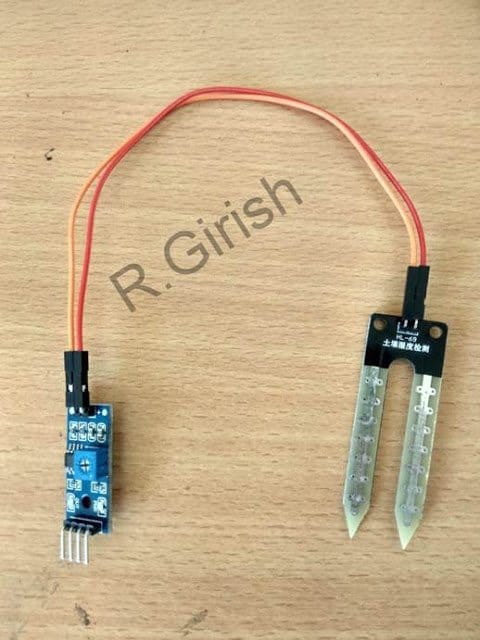
It has 4 + 2 pins, 2 pins for electrode connection and rest of the 4 pins are Vcc, GND, digital output and analog output. We are going to use only the analog output pin for sensing soil moisture.
Since we are not using digital output pin, we will not be using on-board trimmer resistor to calibrate the sensor.
Now, that concludes the soil moisture sensor.
Schematic diagram:
The circuit is kept fairly simple and beginner friendly. The schematic is divided into two parts of the same project to reduce confusion while duplicating the project.
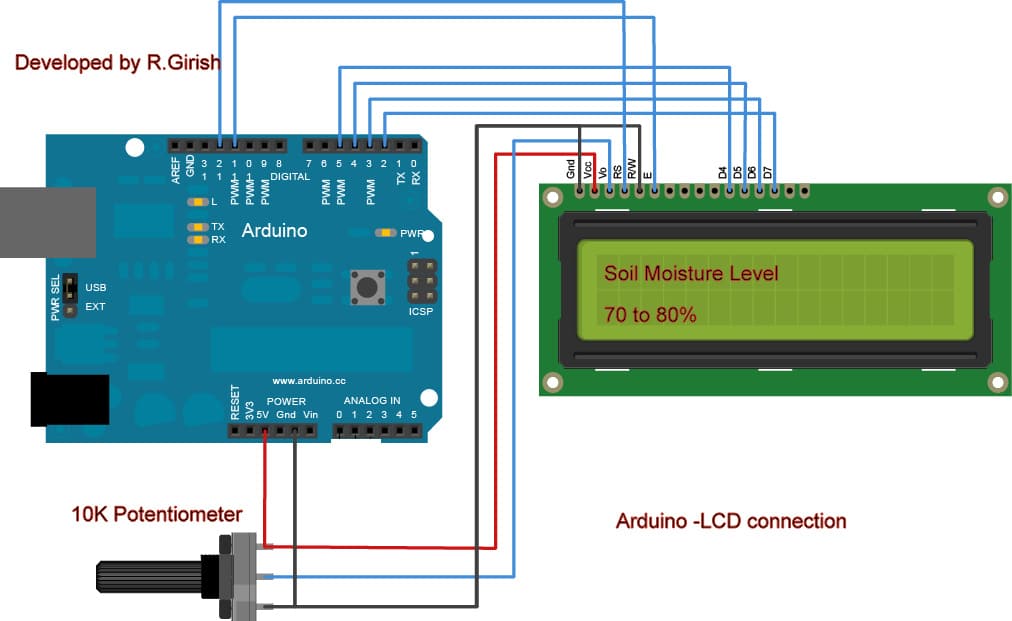
The above schematic is the LCD to arduino wiring. A 10K potentiometer is provided to adjust the contrast of the LCD display.
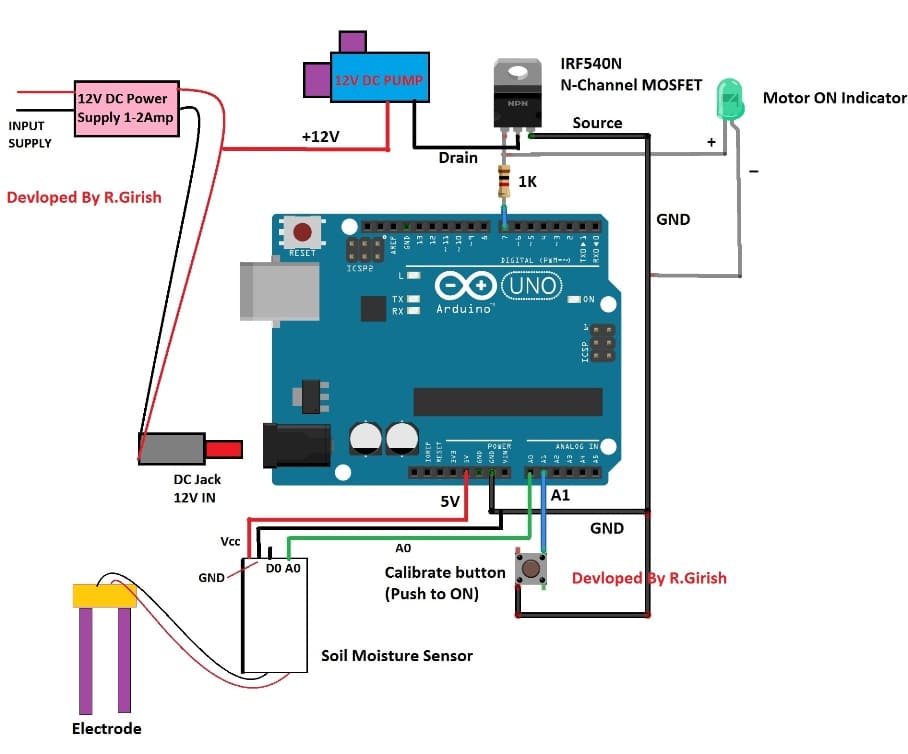
Here is the rest of the schematic consisting of soil moisture sensor, 12V DC pump, a calibrate push button and 12V (1 - 2 amp) power supply. Please use a power supply at least greater than 500mA of 12V DC pump’s current rating.
The MOSFET IRF540N (or any equivalent N-channel) is used instead of BJTs to improve the overall power efficiency of the system.
The pump will water you small garden, make sure you always have adequate amount of water is available.
Program Code:
//-------------Program Developed By R.Girish-------------//
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
int Time = 5; // Set time in minutes
int threshold = 30; // set threshold in percentage 80, 70, 60, 50, 40, 30, 20 only.
int i;
int x;
int y;
int z;
int start;
int calibrateValue;
const int calibrateBTN = A1 ;
const int input = A0;
const int motor = 7;
boolean calibration = false;
boolean rescue = false;
void setup()
{
Serial.begin(9600);
pinMode(input, INPUT);
pinMode(calibrateBTN, INPUT);
pinMode(motor, OUTPUT);
digitalWrite(calibrateBTN, HIGH);
lcd.begin(16,2);
lcd.setCursor(0,0);
lcd.print("Pour water and");
lcd.setCursor(0,1);
lcd.print("press calibrate");
while(!calibration)
{
if(digitalRead(calibrateBTN)==LOW)
{
calibrateValue = analogRead(input);
x = 1023 - calibrateValue;
x = x/10;
Serial.print("Difference = ");
Serial.println(x);
Serial.print("Calibration Value = ");
Serial.println(calibrateValue);
delay(500);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Calibration done");
lcd.setCursor(0,1);
lcd.print("successfully !!!");
calibration = true;
delay(2000);
}
}
}
void loop()
{
if(analogRead(input)<= calibrateValue)
{
delay(500);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 100%");
}
if(analogRead(input) > calibrateValue && analogRead(input) <= calibrateValue+x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 90 to 99%");
}
if(analogRead(input) > calibrateValue+x && analogRead(input) <= calibrateValue+2*x )
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 80 to 90%");
start = 80;
}
if(analogRead(input) > calibrateValue+2*x && analogRead(input) <= calibrateValue+3*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 70 to 80%");
start = 70;
}
if(analogRead(input) > calibrateValue+3*x && analogRead(input) <= calibrateValue+4*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 60 to 70%");
start = 60;
}
if(analogRead(input) > calibrateValue+4*x && analogRead(input) <= calibrateValue+5*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 50 to 60%");
start = 50;
}
if(analogRead(input) > calibrateValue+5*x && analogRead(input) <= calibrateValue+6*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 40 to 50%");
start = 40;
}
if(analogRead(input) > calibrateValue+6*x && analogRead(input) <= calibrateValue+7*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 30 to 40%");
start = 30;
}
if(analogRead(input) > calibrateValue+7*x && analogRead(input) <= calibrateValue+8*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 20 to 30%");
start = 20;
}
if(analogRead(input) > calibrateValue+8*x && analogRead(input) <= calibrateValue+9*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: 10 to 20%");
start = 10;
}
if(analogRead(input) > calibrateValue+9*x && analogRead(input) <= calibrateValue+10*x)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Soil Moisture");
lcd.setCursor(0,1);
lcd.print("Level: < 10%");
rescue = true;
}
if(start == threshold || rescue)
{
y = Time;
digitalWrite(motor, HIGH);
Time = Time*60;
z = Time;
for(i=0; i<Time; i++)
{
z = z - 1;
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("PUMP IS ON, WILL");
lcd.setCursor(0,1);
lcd.print("TURN OFF IN:");
lcd.print(z);
}
Time = y;
rescue = false;
digitalWrite(motor, LOW);
}
delay(1000);
}
//-------------Program Developed By R.Girish-------------//
How to calibrate this automatic irrigation system:
• With completed hardware, insert the electrode on soil, somewhere at the path of water flow.
• Now change the two values in the program 1) The amount of time will take to water all the plants (in minutes). 2) Threshold level below which the arduino triggers the pump. You can set the percentage values 80, 70, 60, 50, 40, 30, 20 only.
int Time = 5; // Set time in minutes
int threshold = 30; // set threshold in percentage 80, 70, 60, 50, 40, 30, 20 only.
Change the values in the program.
• Upload the code to arduino and power the circuit. It will display “pour water and press calibrate”. Now you have to manually water your garden to sufficient level.
• After watering the garden, press the calibrate button. This will determine the conduction of electricity in fully moisture soil and snap shot the reference value.
• Now the system is ready to serve your small garden. Please try to add a power backup for this project. When the power fails the reference calibrated value will be wiped out of memory and you will have to calibrate the system again.
Author’s prototype:
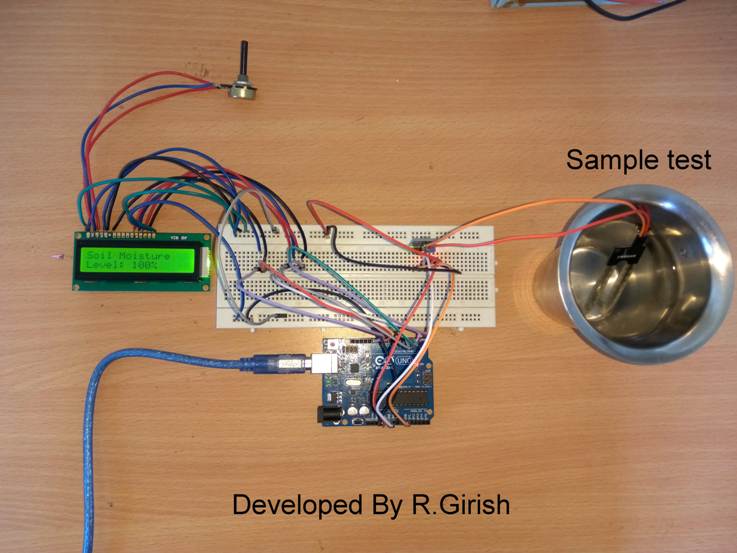
Indication of soil moisture level:
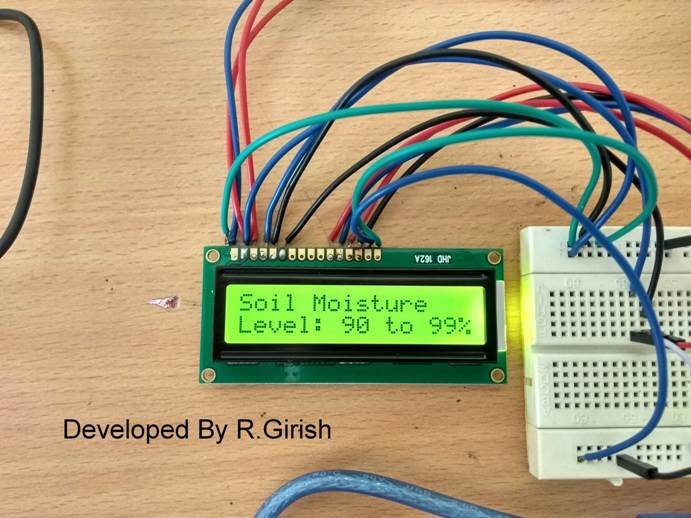
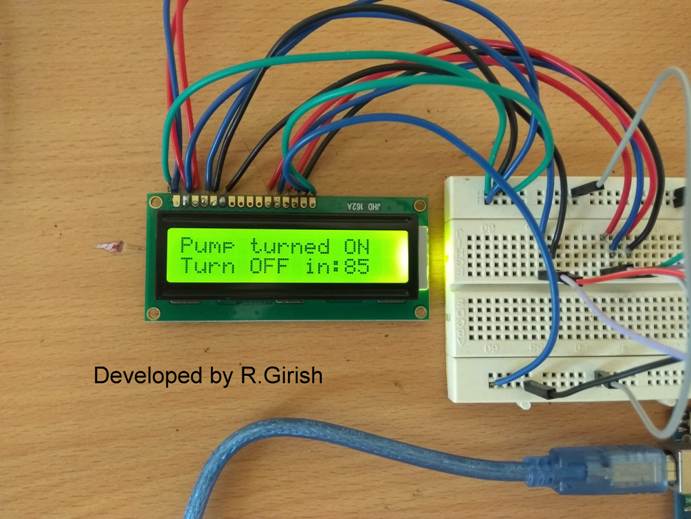
Once the pump is turned ON, it will display remaining time to turn off (in seconds).
can u make one using arduino nano
Hallo sir
I need to control an irrigation pump with three different solenoids
Each time the pump is ON the relevant solenoid valve must be ON and a message to indicate the same.
Hello Francis, code customization is a premium offer from us, presently we are charging Rs.10/- per line of customized code.
Hello sir, i need help regarding a power supply, i was trying to reuse an old ups transformer and was able to change ac to dc but the problem is its voltage fluctuates a lot according to input voltage and here in our area voltage fluctuation is a common problen,eg if the jnput voltage is 180v it supplies 8v DC and if inpur voltage is 250v it supplies 16v dc, what i need is a constant 12v output to drive some 20w leds, is it possible to get a constant output with such fluctuating input voltage
Hello Ishaan,
you can use any suitable voltage regulator IC for correcting the issue, such as this, or any similar
https://www.homemade-circuits.com/2013/04/12v-5-amp-fixed-voltage-regulator-ic.html